How to Build HTML Drag and Drop in Angular
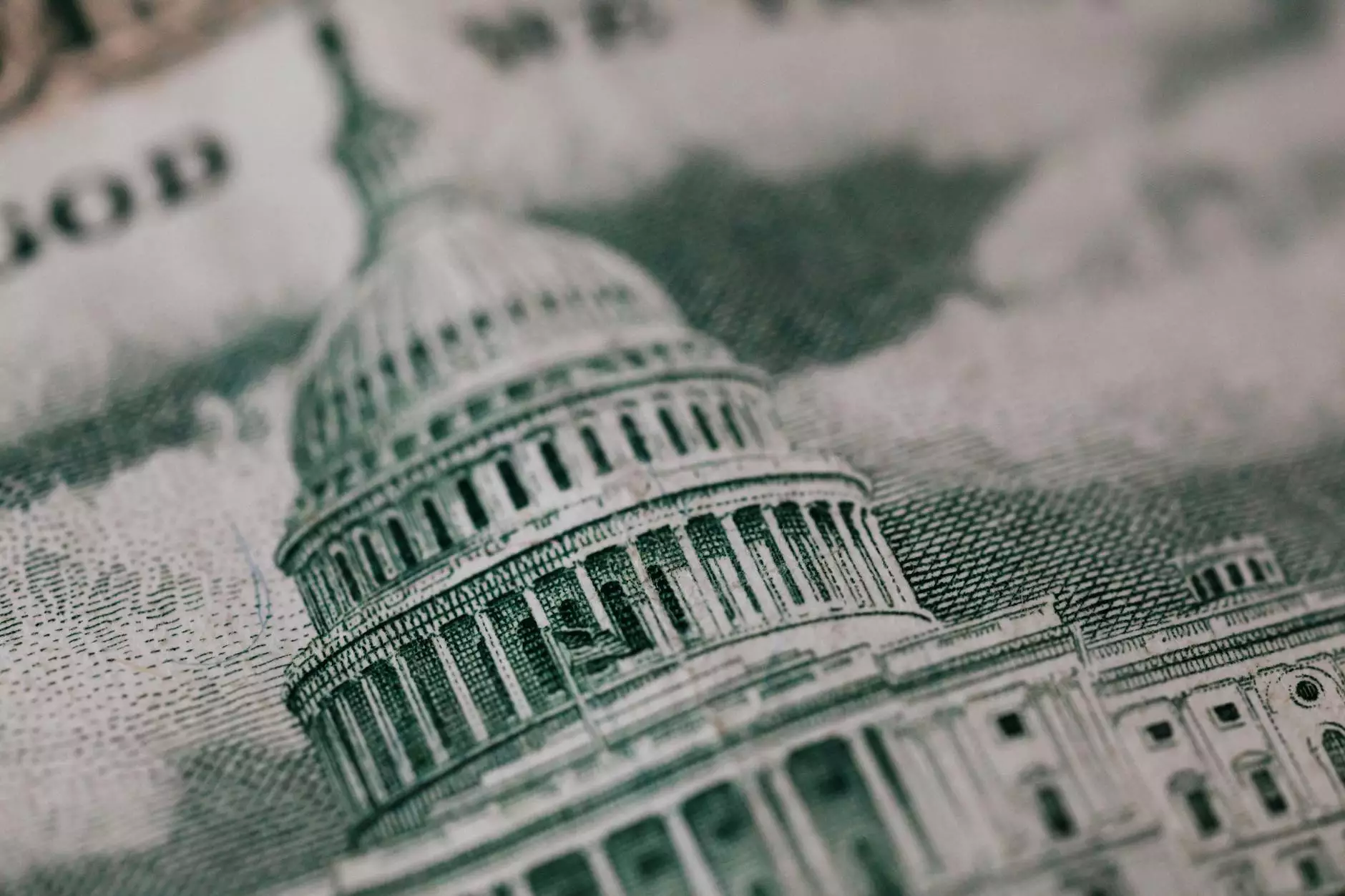
Introduction
Welcome to Seodigitz.com, where we share insights and tutorials on various web development topics. In this article, we will guide you through the process of building HTML drag and drop functionality in Angular.
What is HTML Drag and Drop?
HTML Drag and Drop is a feature that allows users to interact with and manipulate elements on a web page by dragging them from one location to another. It provides a user-friendly way to organize and rearrange content, making web applications more intuitive and engaging.
Getting Started
Before we dive into the implementation, ensure that you have a basic understanding of Angular and its components. If you're new to Angular, we recommend checking out the official Angular documentation for an overview.
Step 1: Setting up the Angular Project
To get started, create a new Angular project or use an existing one. Open your preferred command-line interface and run the following command to generate a new Angular project:
ng new my-drag-drop-projectStep 2: Installing Dependencies
Once the project is set up, navigate into the project directory and install the necessary dependencies. In this example, we will be using the HTML5 drag and drop API, so there is no need for additional libraries or plugins.
cd my-drag-drop-project npm installStep 3: Creating the Drag and Drop Component
Next, let's create a new component that will handle the drag and drop functionality. In your Angular project, run the following command to generate a new component:
ng generate component drag-dropStep 4: Implementing Drag and Drop Functionality
Now it's time to implement the drag and drop functionality in the newly created component. Open the drag-drop.component.ts file and import the necessary modules:
import { Component } from '@angular/core';Inside the component class, define the necessary properties and methods to handle the drag and drop events:
@Component({ selector: 'app-drag-drop', templateUrl: './drag-drop.component.html', styleUrls: ['./drag-drop.component.css'] }) export class DragDropComponent { dragItems: string[] = ['Item 1', 'Item 2', 'Item 3']; onDragStart(event: DragEvent, item: string) { // Handle the drag start event } onDragOver(event: DragEvent) { // Handle the drag over event } onDrop(event: DragEvent) { // Handle the drop event } }In the drag-drop.component.html file, add the necessary HTML markup to display the draggable items and handle the drag and drop events:
{{ item }}Step 5: Styling the Drag and Drop Component
To enhance the user experience and provide visual feedback during drag and drop, it's important to add some styles to the drag and drop component. Open the drag-drop.component.css file and apply the desired styles:
.drag-container { display: flex; } .drag-container div { padding: 10px; margin: 10px; background-color: #f2f2f2; cursor: move; }Conclusion
Congratulations! You have successfully built HTML drag and drop functionality in Angular. By leveraging the HTML5 drag and drop API, you can create interactive and intuitive applications that enhance the user experience. Feel free to explore additional features and customize the drag and drop component based on your specific requirements.
If you need professional assistance with SEO services in Nelligen, Eurobodalla, New South Wales, Australia, or Paxton, Cessnock, New South Wales, Australia, look no further than Seodigitz. We are a leading SEO company specializing in delivering top-notch SEO solutions to businesses in various industries. Contact us today to boost your online presence and outrank your competitors!